Handling XML response with or without namespace in Postman
While testing an XML response in postman, we need to convert XML data to JSON in order to traverse child nodes of an XML document. XML documents can be with/without namespaces. If XML documents have namespaces, then those are declared as attributes to the XML tag. There may or may not be prefixes associated with XML tags containing namespaces. There are different methods or ways to convert XML to JSON, a few of which are described in this blog.
- XML to JSON without namespaces -- using xml2Json
- XML to JSON with namespaces -- using xml2js
Postman version: 8.0.7
Libraries: xml2Json, xml2js
XML to JSON without namespaces
Let's assume we are getting the below XML response from Webservice.console.log(JSON.stringify(responseJson));//logging to postman console
The JSON converted data will be logged in the Postman console as below:
If we want to log the value of city under the address element of second Account.
const responseJson = xml2Json(pm.response.text());//fetch xml data in
text
const city=responseJson.ROOT.Account[1].address.city;//fetch the
desired city value
console.log(city);//logging to postman console
Postman console will log the value as "City2".
In the above example, XML tag does not contain any namespaces. What if XML elements have namespaces and have different namespaces at each level of hierarchy. The next section gives various tips and tricks that can be used to tackle such scenarios.
XML to JSON with namespaces
Let's take another example of XML response which contains namespaces and prefixes.
If we use the same snippet as used in previous example i.e.
console.log(JSON.stringify(responseJson));//logging to postman console
Then output from console will look like.

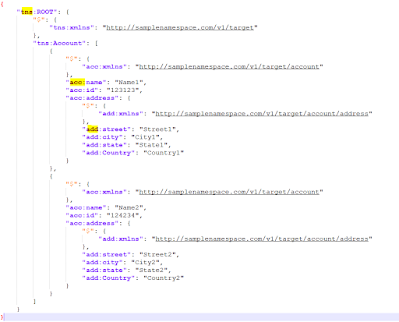

const city=responseJson["tns:ROOT"]["tns:Account"][1]["acc:address"]["add:city"];
console.log(city);
In the above case, we have to explicitly define the key to extract data for each element/node, which will not be easy in most cases. As there can be a possibility, prefix changes wherein instead of tns, ns1 comes, etc. Then above logic will correspond to an invalid object.
Another approach is to convert the XML with namespaces to a form where there are no namespaces. The output of XML response as if there are no namespaces involved. For example:
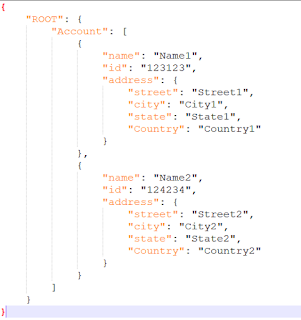
#2 For this, we will be using the "xml2js" library in Postman.
Sample code snippet:
const parseString = require('xml2js').parseString;
const stripPrefix = require('xml2js').processors.stripPrefix;
parseString(respBodyXML, {
tagNameProcessors: [stripPrefix], //removing prefix
ignoreAttrs: true, //ignoring all attributes including xmlns
explicitArray: false,
explicitRoot: true//including root tag inside the JSON
}, function (err, result) {
console.log(JSON.stringify(result));
});
Console Output:
These are some of the ways by which we can handle XML requests inside Postman.
Nice post! This is a very nice blog that I will definitively come back to more times this year! Thanks for the informative post. download Postman
ReplyDelete